Build a WordCloud Visual Using Python and Keyword Extractor APIs
WordCloud is the most popular visualization format to present the most frequent keywords from a specific object. It’s helpful on machine learning, SEO, and social sentiment dataset generation. In this piece, I would walk through how to generate a WordCloud from a list dataset using Python.
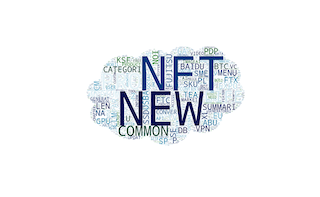
WordCloud is the most popular visualization format to present the most frequent keywords from a specific object. It’s helpful on machine learning, SEO, and social sentiment dataset generation. In this piece, I would walk through how to generate a WordCloud from a list dataset using Python.
Table of Contents: Build a WordCloud Visual Using Python and Keyword Extractor API
- Install Related Python Packages
- Prepare a PNG iamge
- Arraywise the image mask
- Convert the Array Data type into Strings
- Decorate and output the WordCloud image
- Show the WordCloud image
- Full Python Script of WordCloud using BuyfromLo Keyword Extractor (Text, Image KW Extractor APIs)
Install Related Python Packages
For interacting with keyword extractor and outputting WordCloud photo, we need 5 main packages as follows:
from wordcloud import WordCloud
from matplotlib import pyplot as plt
from PIL import Image
import requests
import numpy as np
Prepare a PNG image
Frequent keyword visualization can be engaging with audiences using different shape of photos. The photos can fit in the presentation for the purpose to better convey the data insights.
Thus, before building a wordcloud, we need to select an image in PNG format, which can be filled with keywords later on.
Arraywise the Image Mask
Raw materials are ready. First thing first, we use Pillow to process the image, that includes setting the colors, size, and color mode. Here are the code sample as follows:
icon = Image.open('imageMask2.png')
image_mask = Image.new(mode='RGB', size=icon.size, color=(255,255,255))
image_mask.paste(icon, box=icon)
# Convert an image object to an array ##
rgb_array = np.array(image_mask)
print(rgb_array.shape)
Then, we need to array wise the image mask strings using Numpy.
Import a list of keywords from the target content
Image materials and array dataset are ready. Now, we need to import a list of keywords you aim to build a WordCloud. Here is the keyword extractor API and you can generate top 100 frequent keywords from the content you plugin.
https://www.buyfromlo.com/api/keyword-extractor
If you need to scrape the article content and social content before applying them in keyword extractor, please check out BUYFROMLO Scraper APIs
https://www.buyfromlo.com/api?allType=Scraper
Convert the List Data type into Strings
For WordCloud Python package, they can’t read list datatype. Thus, we need to convert the keyword list into strings using join method like a full article stored in txt file. Here is the code sample as follows:
stringSmartHome = (" ").join(whole_frequent_words)
Decorate and output the WordCloud
We’re almost there and here it’s time to decorate the WordCloud using WordCloud class.
In the WordCloud class, there are several arguments we need to deploy. Here is a list
- Mask: Import the image mask array dataset
- background_color : Set up the WordCloud background color
- Max_words: Define the amount of keywords showing in the WordCloud photo
- Colormap: color tone
- Max_font_size: keyword font size
Here is the sample code as follows:
word_cloud = WordCloud(mask=rgb_array, background_color='white', max_words=8000, colormap='ocean', max_font_size=300).generate(stringSmartHome.upper())
Show the WordCloud image
Last but not least, we use matplotlib to output the photos
plt.imshow(word_cloud, interpolation='bilinear')
plt.axis('off')
plt.show()
Full Python Script of WordCloud using BuyfromLo Keyword Extractor
If you are interested in full python scripts oBuild a WordCloud Visual Using Python and Keyword Extractor APIs, please subscribe to our newsletter by adding the message ‘WordCloud + Full scripts and scraping API free token. We would send you the script when the up-to-date app script is live.
I hope you enjoy reading Build a WordCloud Visual Using Python and Keyword Extractor APIs. If you did, please support us by doing one of the things listed below, because it always helps out our channel.
- Support and Donate to our channel through PayPal (paypal.me/Easy2digital)
- Subscribe to my channel and turn on the notification bell Easy2Digital Youtube channel.
- Follow and like my page Easy2Digital Facebook page
- Share the article on your social network with the hashtag #easy2digital
- You sign up for our weekly newsletter to receive Easy2Digital latest articles, videos, and discount codes
- Subscribe to our monthly membership through Patreon to enjoy exclusive benefits (www.patreon.com/louisludigital)